I have a simple form in which I add an OpenGLWidget, and then set my "MyOpenGLWidget" class to the Promoted Class,
it is successfully created, but is not displayed.
Its constructor is called, but the initializeGL, resizeGL, and paintGL functions are not called.
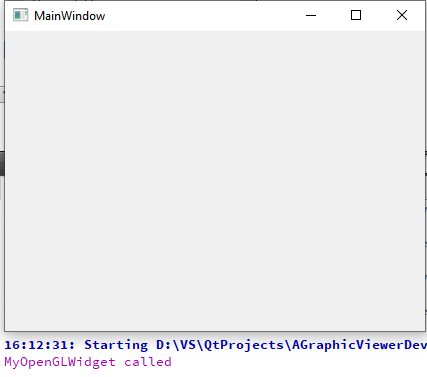
Here is all my code:
main.cpp
#include "mainwindow.h"
#include <QApplication>
int main(int argc, char *argv[])
{
QApplication a(argc, argv);
MainWindow w;
w.show();
return a.exec();
}
mainwindow.cpp
#include "mainwindow.h"
#include "ui_mainwindow.h"
MainWindow::MainWindow(QWidget *parent) : QMainWindow(parent), ui(new Ui::MainWindow)
{
ui->setupUi(this);
}
MainWindow::~MainWindow()
{
delete ui;
}
ui_mainwindow.h
class Ui_MainWindow
{
public:
QWidget *centralwidget;
MyOpenGLWidget *openGLWidget;
void setupUi(QMainWindow *MainWindow)
{
if (MainWindow->objectName().isEmpty())
MainWindow->setObjectName(QString::fromUtf8("MainWindow"));
MainWindow->resize(420, 300);
centralwidget = new QWidget(MainWindow);
centralwidget->setObjectName(QString::fromUtf8("centralwidget"));
openGLWidget = new MyOpenGLWidget(centralwidget);
openGLWidget->setObjectName(QString::fromUtf8("openGLWidget"));
openGLWidget->setGeometry(QRect(60, 50, 300, 200));
MainWindow->setCentralWidget(centralwidget);
retranslateUi(MainWindow);
QMetaObject::connectSlotsByName(MainWindow);
} // setupUi
void retranslateUi(QMainWindow *MainWindow)
{
MainWindow->setWindowTitle(QCoreApplication::translate("MainWindow", "MainWindow", nullptr));
} // retranslateUi
};
namespace Ui {
class MainWindow: public Ui_MainWindow {};
} // namespace Ui
QT_END_NAMESPACE
mainwindow.h
#ifndef MAINWINDOW_H
#define MAINWINDOW_H
#include <QMainWindow>
QT_BEGIN_NAMESPACE
namespace Ui { class MainWindow; }
QT_END_NAMESPACE
class MainWindow : public QMainWindow
{
Q_OBJECT
public:
MainWindow(QWidget *parent = nullptr);
~MainWindow();
private:
Ui::MainWindow *ui;
};
#endif // MAINWINDOW_H
myopenglwidget.cpp
#include "myopenglwidget.h"
#include "globalvars.h"
#include "gl/GLU.h"
MyOpenGLWidget::MyOpenGLWidget(QWidget* parent)
{
qInfo() << "MyOpenGLWidget called";
}
MyOpenGLWidget::~MyOpenGLWidget()
{
qInfo() << "~MyOpenGLWidget called";
}
void MyOpenGLWidget::initializeGL()
{
qInfo() << "initializeGL called!";
initializeOpenGLFunctions();
globalOpenGLContext = this->context();
glClearColor(0.0, 0.0, 0.0, 0.0);
glMatrixMode(GL_PROJECTION);
glLoadIdentity();
gluOrtho2D(-this->width() / 2, this->width() / 2, -this->height() / 2, this->height() / 2);
glViewport(0, 0, this->width(), this->height());
}
void MyOpenGLWidget::resizeGL(int w, int h)
{
qInfo() << "resizeGL called!";
glMatrixMode(GL_PROJECTION);
glLoadIdentity();
gluOrtho2D(-w / 2, w / 2, -h / 2, h / 2);
glViewport(0, 0, w, h);
}
void MyOpenGLWidget::paintGL()
{
qInfo() << "paintGL called!";
glClear(GL_COLOR_BUFFER_BIT | GL_DEPTH_BUFFER_BIT);
glColor3f(1, 1, 1);
glPushMatrix();
glBegin(GL_POLYGON);
glVertex3f(-100, 100, 0);
glVertex3f(100, 100, 0);
glVertex3f(100, -100, 0);
glVertex3f(-100, -100, 0);
glEnd();
glPopMatrix();
update();
}
myopenglwidget.h
#ifndef MYOPENGLWIDGET_H
#define MYOPENGLWIDGET_H
#include <QDebug>
#include <QOpenGLWidget>
#include <qopenglfunctions_3_3_core.h>
#include <gl/GLU.h>
class MyOpenGLWidget : public QOpenGLWidget, protected QOpenGLFunctions_3_3_Core
{
Q_OBJECT
public:
MyOpenGLWidget(QWidget *parent = nullptr);
~MyOpenGLWidget();
protected:
void initializeGL();
void resizeGL(int w, int h);
void paintGL();
};
#endif // MYOPENGLWIDGET_H
question from:
https://stackoverflow.com/questions/65938085/my-qopenglwidget-is-being-created-but-it-doesnt-work